Creating HTTP Web Server in Go
I recently started learning Go, as my choice for backend development & DevOps tooling. I find Go quite similar to JavaScript (as in syntax) & It is going good so far. In order to avoid the trap of tutorial hell, I am adventuring on learn by doing approach.
This is my first project in Go. So here is how to create HTTP web server in Go.
Prerequisite
- Go : Download here
- Code Editor : Of your choice
Setup a module
First, create a new directory for your project and initialize a Go module using the following command:
mkdir webserver
go mod init webserver
You can keep replace webserver with the any desired name for your module.
Create main.go
Navigate to webserver directory & create main.go file.
cd webserver
touch main.go
Import the packages
Now launch your code editor & in your main.go file, import the required packages for building an HTTP server:
package main
import (
"fmt"
"log"
"net/http"
)
Implement the main function
Create the main function to start the server and register the handler function to handle requests:
func main() {
fileServer := http.FileServer(http.Dir("./static"))
http.HandleFunc("/page", anotherPage)
http.Handle("/", fileServer)
fmt.Printf("Starting serving at port 8080")
err := http.ListenAndServe(":8080", nil)
log.Fatal(err)
}
Handler Function
This handler function will handle the request for anotherPage.
func anotherPage(w http.ResponseWriter, r *http.Request){
w.Write([]byte("Welcome to Another Page"))
}
Static Files
Create a new directory at the root named static and create index.html inside it.
mkdir static
touch static/index.html
Populate desired HTML content in the page or copy paste this.
This is how your project directory will look like:
tree .
├── go.mod
├── main.go
└── static
└── index.html
2 directories, 3 files
Run the server
Open a terminal, navigate to the project directory, and run the following command to start the server:
go run main.go
Test the server
Open a web browser and visit http://localhost:8080 (or the desired port you specified in the http.ListenAndServe function). You should see the HTML page displayed. If you visit to http://localhost:8080/page you should see another page reading “Welcome to another page”.
Summary
So that is how we create a basic HTTP web server in Go. It uses functions, routes, handlers, listens & responds to http requests and servers a static HTML file. You can find the entire source code on Github.
That is all for now. I am still in learning phase & I will be sharing more projects in future based on Go.
Reply via mail
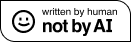